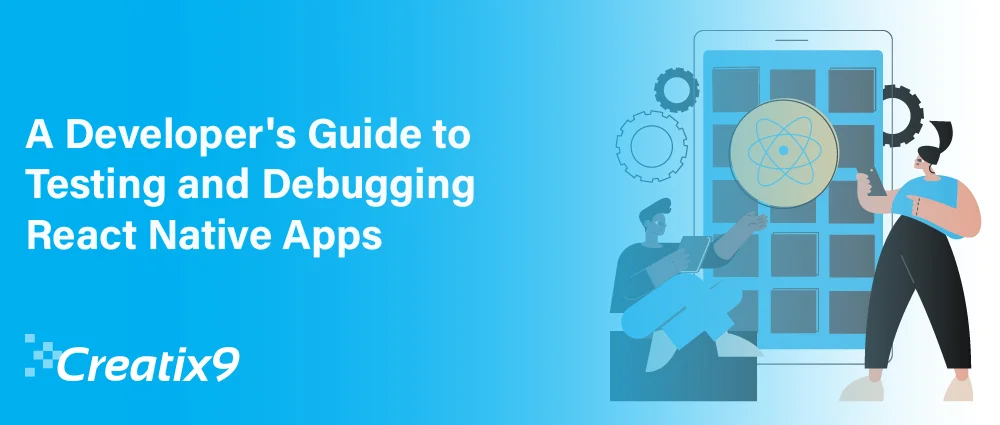
Bugs are an inevitable part of software development, but with the correct technique for testing and debugging, you can make a bug-free zone for your React Native apps. In this blog post, we’ll explore the large-scale process of testing and debugging React Native apps, guaranteeing a smoother react native app development experience and a more reliable last product. Let’s start!
The Importance of Testing and Debugging
Testing and debugging are two cornerstones of prosperous app development. Here’s why they are crucial:
- Reliability and Stability: Testing promises that your app behaves as expected under numerous conditions like user interactions, varying device types, network fluctuations, and diverse operating environments. While debugging helps you spot and fix obstacles that may occur during runtime. By carefully testing and debugging your app, you better its reliability and stability, leading to enhanced customer experience.
- Early Bug Detection: Catching bugs early in the development cycle decreases the time and effort required to fix them. This prevents minor obstacles from snowballing into significant obstacles afterwards, saving either time or resources.
- Cost Efficiency: Fixing bugs and obstacles after the app launch can be costly and harm your brand’s reputation. Timely dealing with bugs prevents possible financial losses and preserves a positive customer perception.
- User Satisfaction: Apps riddled with bugs can frustrate users, prominent to adverse reviews, uninstall, and decrease customer engagement. Robust testing and debugging lead to a smoother customer experience, finally growing customer satisfaction and retention.
Setting Up a Testing Environment
Configuring a correct testing environment is crucial to promise efficient testing procedures. You can go for multiple testing frameworks, have a test suites, and perform unit tests.
Jest and Enzyme are widespread preferences for testing React Native apps. Jest is a powerful testing framework that simplifies unit testing, Jest is like a tool for checking if your JavaScript code is correct. You can use it to write tests in an easy and friendly way. It quickly shows you if your code works as expected. While Enzyme is a tool that helps you test react components by providing helpful functions, just like how React Testing Library does.
A test suite typically includes many test cases that validate different features of your code, assuring that it operates as intended and meets the necessary specifications. Each test within a suite efforts on a particular aspect of your app’s functionality.
Lastly, you can have unit testing and isolate individual components to ensure expected app operations. Unit tests are like little quality checks developers create to guarantee each app works properly.
Rather than testing the entire app at once, unit tests emphasis on testing small code pieces, usually single functions or components. These tests create different situations as if they were happening, and they make sure the code acts exactly how it’s supposed to in those situations.
Automated Testing
Automated testing streamlines the testing process and maintains code quality. You can use continuous Integration (CI). CI tools like “Jenkins” or “GitHub Actions.” These tools automatically run your test suite whenever code changes are pushed to the repository. This instantaneous feedback accelerates the development process and decreases the chances of integrating faulty code.
You can also perform integration tests like jest, Detox, and cypress. These tests check how different parts of your app perform together. Automated integration tests guarantee that new changes don’t break present functionality.
Looking for a React Native app development in the USA? Let our expert digital services provider’s take the lead. With automated testing using Jest, Detox, and Cypress, we ensure top-notch app quality with proper testing and debugging. Build and deploy with confidence using our mobile app development software service. Reach out to us today.
Manual Testing
Manual testing improves automated testing by offering a human perspective. To ensure thorough testing, you can make large-scale test cases covering different scenarios (positive, negative, edge cases). Imagine you’re working on a React Native e-commerce app with a purchasing cart functionality. Here’s how you can make efficient test cases for the cart:
- Positive: Ensure integrating an item into the cart operations correctly. Or Verify updating a product’s quantity and updating the total price.
- Negative: Check if integrating an item with an invalid quantity shows an error. Or Confirm eliminating a non-existent item doesn’t cause errors.
- Edge: Test integrating an oversized item quantity lacking UI issues.
Since React Native apps aim at multiple devices and screen sizes, you can have manual testing across numerous devices to guarantee routine customer experiences.
UI Testing with Detox
As mentioned earlier, Detox is used for automated integration testing. It’s particularly good for testing how your app’s user interface (UI) works. It pretends to be a customer using your app and checks how it responds. For example, it can move through different screens, interact with buttons and other parts of the UI, and ensure everything works as expected.
Detox uses a combination of JavaScript and special code that works closely with your app’s core. You can make Detox act like a customer by tapping buttons, scrolling, and typing text. These tests are important because they ensure your app’s UI works correctly in various situations.
Debugging Tools and Techniques
Effective debugging is essential for identifying and fixing difficulties in your React native app’s code.
For that, you can use a React Native Debugger. This tool is a standalone app that delivers a loyal environment for debugging React Native apps. It provides a debugger interface and allows real-time inspection of components and their states.
Imagine you’re developing a news app, and articles are trying to display correctly on certain devices. The article layout appears inaccurate, and the images are not aligned as expected. This matter may arise from inconsistent styling or device-specific rendering. Use React Native Debugger to review component styles and layouts to ensure consistency across devices and screen sizes.
You can also go for Chrome DevTools for debugging React Native apps operating in the Chrome browser. It enables you to inspect the React component hierarchy, examine network requests, and debug JavaScript code.
Sometimes app suddenly freezes or crashes after a specific action, like submitting a form. The issue can be an infinite loop triggered by incorrect state management. In such cases, tools like Chrome DevTools and React Native Debugger are valuable for establishing breakpoints in the suspected code sections. You can identify and correct improper state updates or loop conditions that may cause infinite loops. Ensure correct termination through testing.
Safari Web Inspector is also a good option for debugging on iOS devices. Safari provides a Web Inspector feature that enables developers to debug web information and hybrid apps remotely.
You can go for UXCam as it track the efficiency of your React native app. It support indirectly with testing and debugging React Native applications. It keeps track of user sessions to display how users engage with your software. This can aid in locating problems and offer information for debugging. It’s also helpful for testing because you can see actual or simulated user interactions and see how effectively your app works from the user’s point of view.
For physical devices, you can also permit remote debugging. This enables you to link your device to a development machine and use these debugging tools from your computer while the app runs on the device.
Logging and Error Handling
Proper logging and error management raise your capability to track and address issues. You can implement routine and well-informed logging throughout your codebase. This supports trace your app’s flow and detect difficulties via clear record of events.
Logging is the practise of recording vital data, actions, and errors that occurs in a software app during usage. It’s like preserving a record of what the app is performing. Logging benefits developers to learn apps behaves, find and fix bugs, and scan its performance.
It’s vital for debugging, monitoring, and improving the react native app quality. In a React Native app, you use operations like “console.log()” to make log messages to get insights into what’s happening in your app.
You can build custom error messages with relevant data like the component name, activity performed, and state data to make debugging faster and effective.
Reproducing and Isolating Bugs
Efficient debugging starts with being capable of reproducing the issue consistently. Document the steps or conditions that lead to the bug’s occurrence. This lets you and your group experience the bug firsthand, which is crucial for efficient debugging.
Once reproduced, isolate the bug by gently simplifying the code and eliminating unnecessary components or features. This helps narrow down the root reason and prevents distractions from unrelated code.
Use tools like React Native Flipper to reproduce and Isolating Bugs.
Version Control and Debugging
Version control systems like Git are invaluable during debugging. When you find a bug, you can use Git to revert to a working edition and then progressively apply changes to recognise where the bug exists.
Git bisect is a feature that automatically identifies the commit where a bug was introduced. By marking a recognised good commit and a recognised bad commit, Git bisect helps you efficiently narrow down the problematic code.
Collaborative Debugging
Debugging is often a collaborative effort. Discuss the bug with your group members, share insights, and leverage diverse perspectives to uncover the root cause. Two heads are often better than one when it arrives to debugging intricate issues.
VS Code Live Share is a real-time collaboration tool enabling group members to debug jointly. You can share your debugging session, breakpoints, and code changes, making it easier to perform together, especially in distributed teams.
Conclusion
Remember, debugging is not always straightforward, and sometimes obstacles may possess multiple contributing factors. Patience and a systematic technique are essential. Break down the problem, isolate possible causes, and test your assumptions. Each debugging situation you encounter hones your skills and contributes to your knowledge as a React Native developer.
Want to hire an organisation to make a React native app free of bugs, contact our React native app development company. From ideas to reality, we design React Native apps that improve your brand’s digital presence.
As a top digital services provider, we’re present to help you with digital services from mobile app development software to its marketing. Contact us now!